Predicting Bitcoin Prices Using Machine Learning Techniques
Written on
Chapter 1: Introduction to Bitcoin Price Prediction
Bitcoin, a cryptocurrency that has garnered significant attention recently, poses a considerable challenge when it comes to forecasting its price due to its inherent volatility. This article will demonstrate how to utilize Python to predict Bitcoin's price using historical data along with Stochastic Gradient Descent (SGD) regression.
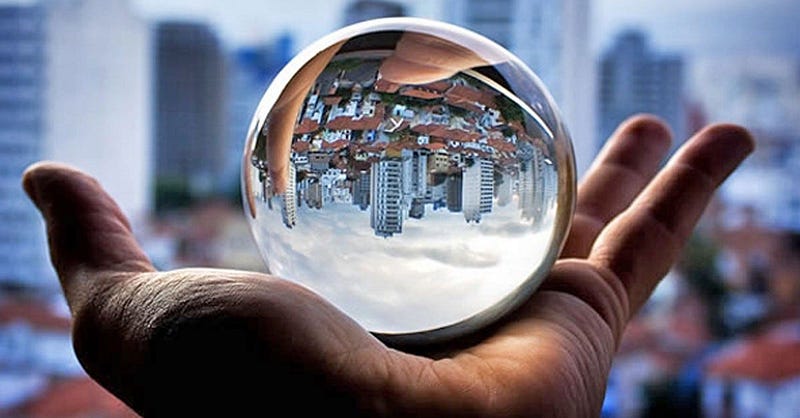
The first task involves importing the essential libraries. The libraries we will employ include Pandas, NumPy, Scikit-learn, and TA-Lib—a technical analysis toolkit that aids in calculating necessary indicators.
import pandas as pd
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.linear_model import SGDRegressor
from sklearn.preprocessing import StandardScaler
import ta
import pandas_ta as pda
Next, we must load the historical data. For this example, we will utilize daily Bitcoin data, which can be sourced from platforms like Yahoo Finance or Quandl. Here, we will work with a CSV file containing Bitcoin's daily prices.
df = pd.read_csv('BTC1D.csv')
df2 = pd.read_csv('BTC1D.csv')
Following data loading, we need to specify the features for our price prediction model. We will include the Relative Strength Index (RSI), Average Directional Index (ADX), Kaufman Adaptive Moving Average (KAMA), and various Exponential Moving Averages (EMAs) with distinct time frames, all of which are commonly used in technical analysis.
df['RSI'] = ta.momentum.rsi(close=df['close'], window=14, fillna=True)
df['EMA1'] = ta.trend.ema_indicator(close=df['close'], window=9, fillna=True)
df['EMA2'] = ta.trend.ema_indicator(close=df['close'], window=21, fillna=True)
df['EMA3'] = ta.trend.ema_indicator(close=df['close'], window=50, fillna=True)
df['EMA4'] = ta.trend.ema_indicator(close=df['close'], window=100, fillna=True)
df['ADX'] = ta.trend.adx(high=df['high'], low=df['low'], close=df['close'], window=14, fillna=True)
df['KAMA'] = ta.momentum.kama(close=df['close'], window=10, pow1=2, pow2=30, fillna=True)
features = ['close', 'RSI', 'ADX', 'KAMA', 'EMA1', 'EMA2', 'EMA3', 'EMA4']
The target variable, which represents Bitcoin's price for the following day, will be defined by shifting the closing price by one day and omitting the last row, which yields a null value.
target = 'price_1_day'
df['price_1_day'] = df['close'].shift(-1)
df = df.dropna()
Subsequently, we will divide the data into training and testing sets, allocating 20% for testing and the remainder for training the model.
X_train, X_test, y_train, y_test = train_test_split(df[features], df[target], test_size=0.2, random_state=42)
Before model training, it is crucial to scale the data, enhancing performance, especially in neural networks.
scaler = StandardScaler()
X_train_scaled = scaler.fit_transform(X_train)
X_test_scaled = scaler.transform(X_test)
Now, we can create and train the SGDRegressor model.
svr = SGDRegressor(max_iter=100000, tol=1e-3)
svr.fit(X_train_scaled, y_train)
To make predictions on the test set:
y_pred = svr.predict(X_test_scaled)
We can evaluate the model's performance with the following code snippet:
score = svr.score(X_test_scaled, y_test)
print('Accuracy:', score)
The data is now prepared for future predictions. By taking the last entry from the dataset and scaling it, we can predict future Bitcoin prices.
df2 = df2.dropna()
X_future = df2[features].iloc[-1:].values
X_future_scaled = scaler.transform(X_future)
y_future = svr.predict(X_future_scaled)
After executing the above code, we achieve the following output: Accuracy: 0.9263835629844685. This high accuracy score indicates that the model performs remarkably well. The anticipated Bitcoin price for the next day is 27,702.
Additionally, a plot illustrates both the actual and predicted closing prices of Bitcoin over time. The predicted prices generally align with the trend of the actual prices, though some discrepancies exist.
X_future2 = df2[features].values
X_future_scaled2 = scaler.transform(X_future2)
df2['pred'] = svr.predict(X_future_scaled2)
import matplotlib.pyplot as plt
# Plot the actual closing prices
plt.plot(df2['close'], label='Actual Closing Prices')
# Predicted values for the next week
plt.plot(df2['pred'], label='Predicted Closing Prices')
plt.legend()
plt.show()
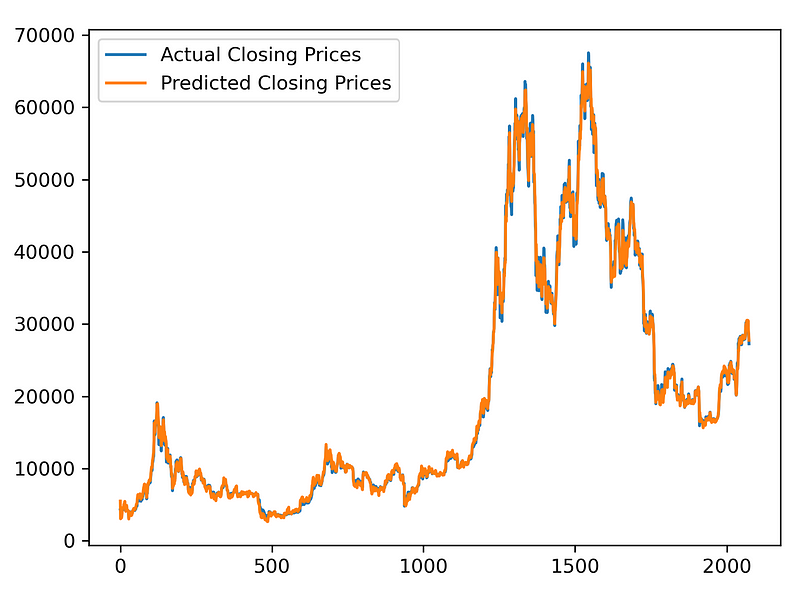
In summary, this code illustrates how Python and machine learning can be leveraged to predict Bitcoin prices based on various technical indicators. It is essential to recognize that this method is just one of many approaches to predicting Bitcoin prices, as numerous factors, including market sentiment and global economic conditions, can influence the price. Thus, it is vital to consider multiple elements rather than relying solely on technical indicators for investment decisions.
A Message from InsiderFinance

Thanks for being a part of our community! Before you go: clap for the story and follow the author. View more content in the InsiderFinance Wire. Take our FREE Masterclass. Discover Powerful Trading Tools.
Chapter 2: Video Insights on Bitcoin Predictions
To further enhance your understanding, check out this informative video on automated price trend lines in Python, which serves as a valuable algorithmic trading indicator.
This video provides in-depth explanations and practical examples to help you grasp the concepts of price prediction better.