Transforming Slack Audio Messages into Text Summaries Using ML
Written on
Chapter 1: Introduction to Audio Summarization
In this article, we will explore how to turn audio conversations from Slack into written summaries. Slack enables users to share audio or video recordings with their colleagues, providing an effective communication tool. There’s a saying I cherish: “A picture is worth a thousand words.” Sometimes, articulating an idea in words can be quite difficult or time-consuming. This is where audio and video messages truly shine.
As a technology enthusiast, I wanted to merge this fascinating Slack capability with machine learning. There’s always room for enhancement, and the key lies in how you tackle the challenge and create a solution. My passion for Machine Learning projects stems from their ability to integrate various topics, making learning both enjoyable and lasting.
Let’s dive in!
Table of Contents:
- Getting Started
- Step 1 — Required Libraries
- Step 2 — Recording Audio on Slack
- Step 3 — Uploading Audio to the API
- Step 4 — Transcribing Speech to Text
- Final Step — Saving the Summary
Getting Started
For this project, we will utilize AssemblyAI’s Speech-to-Text API, which is well-optimized for machine learning tasks. It’s free to use, and once you create an account, you’ll receive a unique API key to access its features. AssemblyAI offers robust services, including topic detection and content moderation. For our purposes, we’ll focus on the speech-to-text functionality with an automatic summarization feature, which is ideal for note-taking.
Jupyter Notebook
We will employ Jupyter Notebook as our coding environment. I prefer it for its open-source nature and the ability to execute code in discrete blocks. This feature is particularly beneficial for projects in Data Science and Machine Learning.
Step 1 — Required Libraries
If you have previous experience with machine learning, you’re likely familiar with some Python libraries. If you’re new to this, there’s no need to worry; installation is straightforward. The libraries we’ll use for this project include requests, time, and sys, all of which come pre-installed with Python.
Let’s import these libraries into our program:
import requests
import time
import sys
Step 2 — Recording Audio on Slack
Now, we’ll discuss how to record an audio clip in Slack and download it to our device.
So, what exactly is Slack? It’s a messaging platform designed for businesses that connects users to the information they need. By uniting individuals into one cohesive team, Slack transforms organizational communication.
Slack can be accessed as a web application or via software installed on your device. Below is a screenshot of my personal workspace, which you can set up using your email.
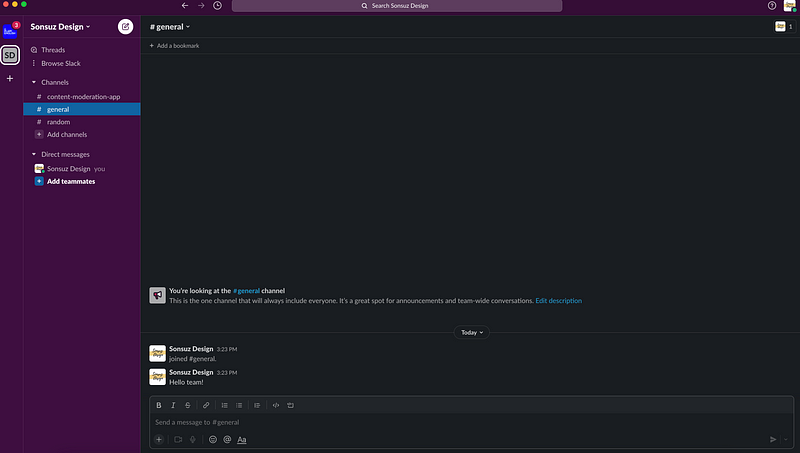
Now, let’s proceed to record an audio clip. This feature is available for free during a 30-day trial. I recorded a message by clicking the microphone icon. In the image below, you can see the options available for audio messages. We'll click on download to use this audio clip for our machine learning program.
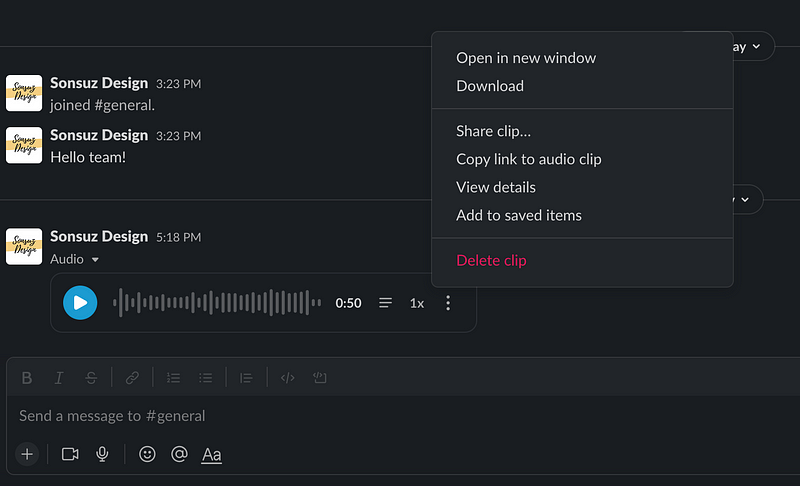
Step 3 — Uploading Audio to the API
Next, we’ll upload the downloaded audio to the cloud, where the Speech-to-Text transcription will take place using AssemblyAI. There’s no additional software to install; we only need our API key.
audio_data = "slack_recording.wav"
def upload_audio(audio_data, chunk_size=5242880):
with open(audio_data, 'rb') as audio_file:
while True:
data = audio_file.read(chunk_size)
if not data:
breakyield data
headers = {
"authorization": "Our API key goes here."
}
audio_data represents the file path of the audio recording. The upload_audio function reads the audio file, and the headers dictionary is where we include our API key. The response variable captures the result of our POST request to the API.
After executing this code block, we can print the response:
print(response.json())
The output should look like this:
Step 4 — Transcribing Speech to Text
We’re almost there! This step involves converting the uploaded audio file into text. We will also utilize the auto-summary feature of the API to receive a condensed version of the audio.
Here’s the code to send our request to the API:
data = {
"audio_url": "the upload url address goes here",
"auto_chapters": "TRUE",
}
headers = {
"authorization": "Our API key goes here.",
"content-type": "application/json"
}
response = requests.post(speech_to_text_api, json=data, headers=headers)
Once we run this code, let’s check the response:
print(response.json())
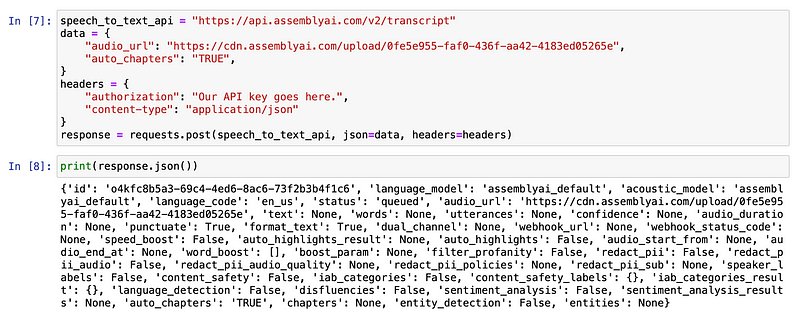
Our request is now queued for processing. We need to note down the ID value from this request to check its status later.
Final Step — Saving the Summary
In this step, we’ll query the API for the status of our earlier request:
headers = {
"authorization": "Our API key goes here."
}
response = requests.get(request_url, headers=headers)
summarized_text = response.json()['chapters']
Here’s the summarized output from my audio message:

Excellent! Let’s save this summary to a text document:
file = open("summary_text.txt", "w")
file.write("Summary of the audio message is as follows: n")
file.write(summarized_text[0]['summary'])
file.close()
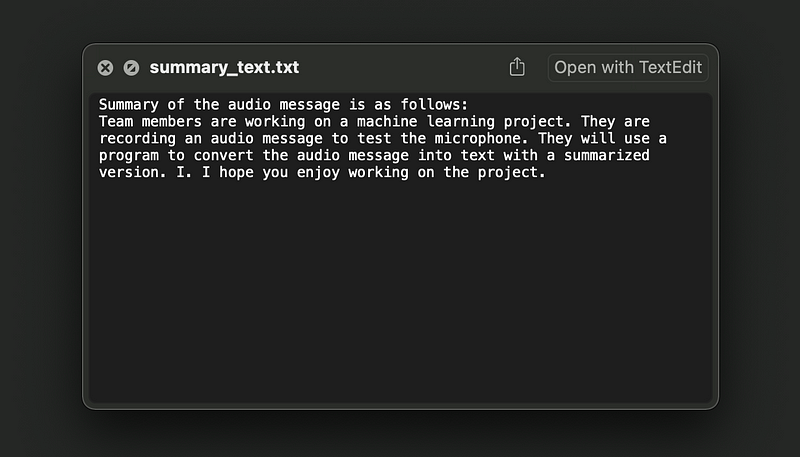
Congratulations! We’ve developed a program capable of summarizing audio messages. The Slack audio feature is just one application of this project. Feel free to experiment with different audio clips and observe how it performs. This is a fantastic way to extract key points from audio content.
It’s inspiring to see how machine learning is applied in practical scenarios — I hope you enjoyed this article and learned something valuable today.
I am Behic Guven, and I’m passionate about sharing insights on programming, education, and life. Subscribe to my content for ongoing inspiration.
If you’re curious about the types of articles I write, here are a few examples:
- Building a Face Recognizer using Python
- Step-by-Step Guide — Building a Prediction Model in Python
- Building a Speech Emotion Recognizer using Python
For more content, visit PlainEnglish.io. Sign up for our free weekly newsletter and follow us on Twitter and LinkedIn. Join our community on Discord.
Chapter 2: Enhancing Meeting Summaries with AI
This video explores how to use AI alongside Slack for effective meeting summaries.
Chapter 3: Streamlining Conversations with AI on Slack
In this video, learn how to leverage Slack AI for summarizing conversations efficiently.