Creating an Effective FAQ Section with HTML: A Comprehensive Guide
Written on
Chapter 1: Introduction to FAQ Sections
Welcome to the 42nd entry in our HTML Tips series! This time, we’ll be exploring how to create a straightforward FAQ (Frequently Asked Questions) section. FAQ sections are vital for websites, offering users quick answers to their inquiries, improving user satisfaction, and minimizing the need for direct support.
In this tutorial, we'll guide you through the process of building an engaging FAQ section using HTML. We will discuss the necessary structure, styling, and additional features that make your FAQ section both functional and user-friendly.
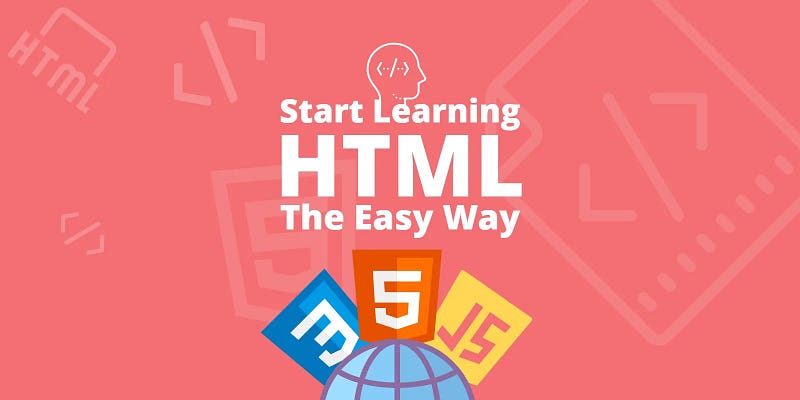
Why Implement an FAQ Section?
An FAQ section is invaluable for users seeking quick answers regarding your website, products, or services. It can:
- Minimize Customer Support Inquiries: By providing answers to common questions, users will be less inclined to reach out for support over simple matters.
- Enhance User Experience: Instant answers can significantly improve the overall experience for visitors on your site.
- Boost Engagement: A well-structured FAQ section keeps users interested by delivering helpful information in a user-friendly layout.
Basic Structure of an FAQ Section
1. Building the HTML Framework
The fundamental HTML layout for an FAQ section usually involves listing questions and their corresponding answers. Below is a basic example demonstrating how to structure an FAQ section:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>FAQ Section Example</title>
<style>
.faq-container {
max-width: 800px;
margin: 0 auto;
padding: 20px;
font-family: Arial, sans-serif;
}
.faq-item {
margin-bottom: 10px;
border-bottom: 1px solid #ccc;
padding-bottom: 10px;
}
.faq-question {
font-weight: bold;
cursor: pointer;
padding: 10px;
background-color: #f7f7f7;
border-radius: 4px;
}
.faq-answer {
display: none;
padding: 10px;
background-color: #f0f0f0;
border-left: 3px solid #007BFF;
border-radius: 4px;
}
.active .faq-answer {
display: block;}
</style>
</head>
<body>
<div class="faq-container">
<div class="faq-item">
<div class="faq-question">What is your return policy?</div>
<div class="faq-answer">Our return policy allows for returns within 30 days of purchase. Items must be in their original condition.</div>
</div>
<div class="faq-item">
<div class="faq-question">How can I track my order?</div>
<div class="faq-answer">You can track your order using the tracking number provided in your confirmation email. Visit our tracking page to enter the number.</div>
</div>
<div class="faq-item">
<div class="faq-question">Do you ship internationally?</div>
<div class="faq-answer">Yes, we offer international shipping to most countries. Shipping fees and delivery times vary depending on the destination.</div>
</div>
</div>
<script>
// JavaScript for toggling FAQ answers
document.querySelectorAll('.faq-question').forEach(item => {
item.addEventListener('click', () => {
item.parentElement.classList.toggle('active');});
});
</script>
</body>
</html>
#### Explanation of the HTML Structure
- faq-container: This section wraps all FAQ items and centralizes them on the page.
- faq-item: Each question-answer pair is represented with a border and padding for separation.
- faq-question: The clickable element that users select to show or hide the answer; styled to resemble a button.
- faq-answer: Initially hidden and revealed when the corresponding question is clicked.
2. Enhancing Accessibility
It’s crucial to ensure that your FAQ section is accessible to all users, including those utilizing screen readers or keyboard navigation. Here are some tips for improving accessibility:
- Utilize ARIA Attributes: Incorporate ARIA roles and attributes to enhance accessibility.
<div class="faq-item" role="region" aria-labelledby="faq1-question">
<div id="faq1-question" class="faq-question" tabindex="0" role="button" aria-expanded="false">What is your return policy?</div>
<div class="faq-answer" aria-hidden="true">Our return policy allows for returns within 30 days of purchase. Items must be in their original condition.</div>
</div>
- Keyboard Navigation: Ensure that users can traverse FAQ questions using their keyboard (e.g., arrow keys).
item.addEventListener('keydown', event => {
if (event.key === 'Enter' || event.key === ' ') {
event.preventDefault();
item.click();
}
});
3. Responsive Design for Various Devices
It's important to make sure your FAQ section is responsive and appears well on all devices by employing responsive design techniques.
@media (max-width: 600px) {
.faq-question {
font-size: 16px;}
.faq-answer {
font-size: 14px;}
}
4. Adding Visual Elements
Consider incorporating icons to your FAQ section for improved visual appeal. For instance, you could use plus and minus icons to indicate the visibility of answers.
<div class="faq-question">
<span class="icon">+</span>
What is your return policy?
</div>
.icon {
margin-right: 10px;
}
.active .icon {
content: "-";
}
5. Advanced Features: Dynamic FAQ Data
For a more sophisticated FAQ section, you can dynamically fetch FAQ data from a server or API. This allows for easier management and updates without altering the HTML code.
document.addEventListener('DOMContentLoaded', () => {
fetch('faqs.json')
.then(response => response.json())
.then(data => {
const faqContainer = document.querySelector('.faq-container');
data.faqs.forEach(faq => {
const faqItem = document.createElement('div');
faqItem.classList.add('faq-item');
const question = document.createElement('div');
question.classList.add('faq-question');
question.textContent = faq.question;
const answer = document.createElement('div');
answer.classList.add('faq-answer');
answer.textContent = faq.answer;
faqItem.appendChild(question);
faqItem.appendChild(answer);
faqContainer.appendChild(faqItem);
});
document.querySelectorAll('.faq-question').forEach(item => {
item.addEventListener('click', () => {
item.parentElement.classList.toggle('active');});
});
});
});
#### Explanation of Dynamic Content
- faqs.json: A JSON file that holds FAQ data.
- Dynamic Content: FAQs are generated and displayed based on the fetched data.
Conclusion
Building a simple HTML FAQ section is a fantastic way to enhance the user experience on your website. By following the steps outlined in this guide, you can create an FAQ section that is both functional and visually appealing.
Incorporate the various elements effectively, improve accessibility, and consider advanced features to make your FAQ section even more beneficial. Whether you are managing static FAQs or dynamic data, this guide equips you with the tools and techniques required to develop a successful FAQ section.
If you have any questions or need further assistance, don’t hesitate to ask. Happy coding!
The video titled "You can do THAT with HTML? | 7 Cool HTML Tricks for Beginners" showcases innovative HTML techniques that can complement your learning. Discover practical tips and tricks to enhance your web development skills!