Mastering Dictionary Iteration with While Loops in Python
Written on
Chapter 1: Understanding Python Dictionaries
A dictionary is one of the fundamental data types in Python. From version 3.7 onward, dictionaries maintain the order of elements, consisting of unique key-value pairs. The keys must be immutable and can be of types such as numbers, strings, or tuples. Each key corresponds to a value, which can be a number (integer, float, or boolean), a string, a list, or even another dictionary. Unlike keys, values are mutable.
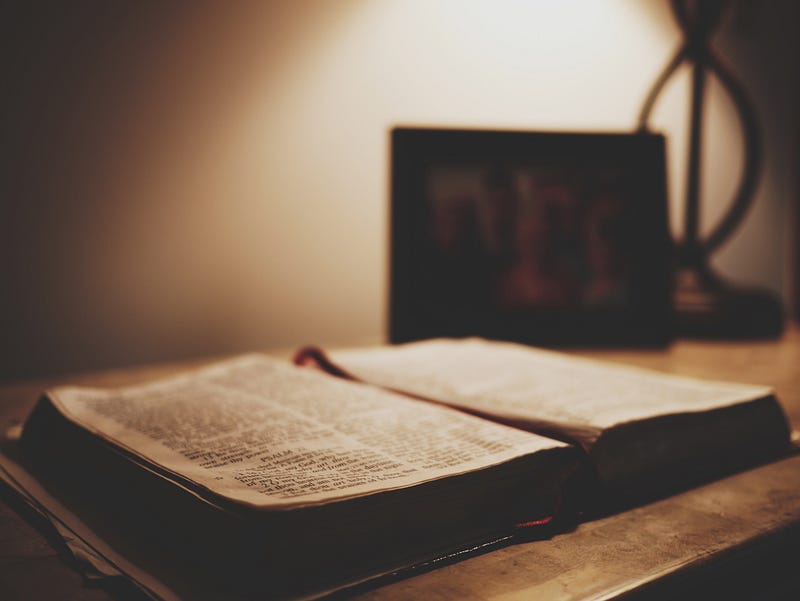
Chapter 2: Utilizing While Loops
A while loop allows the execution of a block of statements as long as a specified condition remains true.
Example of a Dictionary with a While Loop:
Consider the following dictionary:
books = {
"Learning Python": "Mark Lutz",
"Think Python": "Allen B. Downey",
"Fluent Python": "Luciano Ramalho"
}
To access the values, you can iterate through the keys using the indexing operator:
key = list(books) # Converts keys into a list
i = 0
while i < len(key):
print(key[i], ":", books[key[i]])
i += 1
Output:
Learning Python : Mark Lutz
Think Python : Allen B. Downey
Fluent Python : Luciano Ramalho
The Break Statement
The break statement allows you to terminate the loop prematurely, even if the while condition is still true.
Example:
Exit the loop when i is 2:
books = {
"Learning Python": "Mark Lutz",
"Think Python": "Allen B. Downey",
"Fluent Python": "Luciano Ramalho"
}
key = list(books)
i = 0
while i < len(key):
if i == 2:
breakprint(key[i], ":", books[key[i]])
i += 1
Output:
Learning Python : Mark Lutz
Think Python : Allen B. Downey
The Else Statement
An else statement can execute a block of code after the loop finishes if the condition is no longer true.
Example:
Print "The End" when the loop completes:
books = {
"Learning Python": "Mark Lutz",
"Think Python": "Allen B. Downey",
"Fluent Python": "Luciano Ramalho"
}
key = list(books)
i = 0
while i < len(key):
if i == 3:
continueprint(key[i], ":", books[key[i]])
i += 1
else:
print("The End")
Output:
Learning Python : Mark Lutz
Think Python : Allen B. Downey
Fluent Python : Luciano Ramalho
The End
For additional resources, consider exploring our eBook: Solved Exercises Of Python Dictionary.
For more insights, visit PlainEnglish.io. Stay updated by signing up for our free weekly newsletter and follow us on Twitter, LinkedIn, YouTube, and Discord.
Chapter 3: Video Resources
To enhance your learning experience, check out these helpful videos:
This video titled "Using Dictionaries, For Loops and While Loops in Python!" provides a comprehensive overview of working with these essential structures in Python.
Another valuable resource is "For and While Loops and List/Dict Comprehension in Python," which delves deeper into iteration techniques.